Welcome friends, In this blog I am going to talk about Realm Database. It is fast and easy way to create local storage database in Android apps. It is supereasy than the traditional method of creating databases using SQLite.
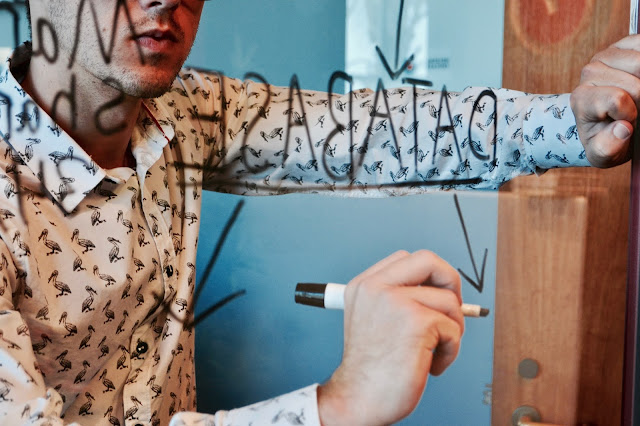
So, without much ado, let’s get started!
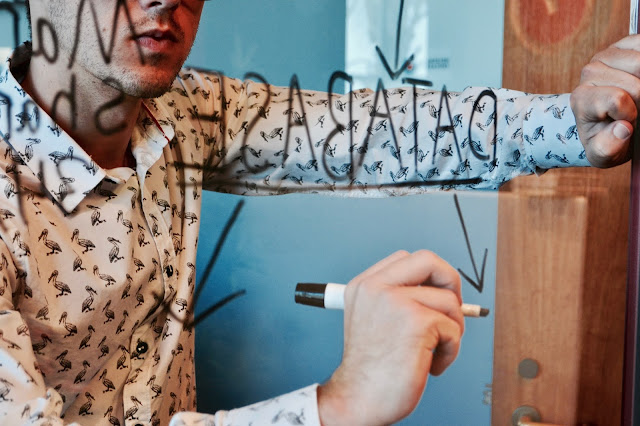
So, without much ado, let’s get started!
1. Installation
Step 1: Add the classpath dependency to the project level
Step 1: Add the classpath dependency to the project level
build.gradle
file.1 2 3 4 5 6 7 8 | buildscript { repositories { jcenter() } dependencies { classpath "io.realm:realm-gradle-plugin:10.0.1" } } |
Step 2: Apply the
realm-android
plugin to the top of the application level build.gradle
file.1
| apply plugin: 'com.android.application' apply plugin: 'realm-android'
|
2. Creating a helper class
To Initialize Realm, you have to call
To do this,
To Initialize Realm, you have to call
Realm.init(context);
just once per application.To do this,
Step 1 : Add
android:name=".MyApplication"
attribute inside application
tag in AndroidManifest.xml
.
Step 2 : Create a new file
MyApplication.java
and paste below code in it.1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | import android.app.Application; import io.realm.Realm; import io.realm.RealmConfiguration; public class MyApplication extends Application { @Override public void onCreate() { super.onCreate(); Realm.init(this); RealmConfiguration realmConfig = new RealmConfiguration.Builder() .name("mydb.realm") .schemaVersion(0) .build(); Realm.setDefaultConfiguration(realmConfig); } } |
3. Create a structure of realm database
Create a new file
Create a new file
RealmBean.java
.1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 | import io.realm.RealmObject; import io.realm.annotations.PrimaryKey; import io.realm.annotations.Required; public class RealmBean extends RealmObject { @Required public String object; @PrimaryKey public String sr; public String getObject() { return object; } public void setObject(String object) { this.object = object; } public String getSr() { return sr; } public void setSr(String sr) { this.sr = sr; } } |
In the above code,
You can add any number of fields you want.
sr
is ‘primary key’ and object
is a ‘required field’.You can add any number of fields you want.
4. Get Instance of Realm
You will need a realm instance to read/write in realm database.
To get a Realm instance, type
You will need a realm instance to read/write in realm database.
To get a Realm instance, type
1
| Realm realm = Realm.getDefaultInstance();
|
write it in
onCreate()
method.
And do not forget to close the realm object when your work is done.
1
| realm.close();
|
write it in
onDestroy()
method.
5. Read data
Use below boilerplate code to read data from the realm database.
Use below boilerplate code to read data from the realm database.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | realm.executeTransaction(new Realm.Transaction() { @Override public void execute(Realm realm) { try { RealmResults<RealmBean> results; results = realm.where(RealmBean.class).findAll(); Log.e("results count : ",results.size()+""); for(int i = 0 ; i < results.size(); i++){ Log.e("result "+i, results.get(i).getObject()); } }catch (Exception e){ e.printStackTrace(); } } }); |
6. Write data
Use below boilerplate code to write data to the realm database.
Use below boilerplate code to write data to the realm database.
1 2 3 4 5 6 7 8 9 10 11 | realm.executeTransaction(new Realm.Transaction() { @Override public void execute(Realm realm) { try { Realm.init(getApplicationContext()); RealmBean object = realm.createObject(RealmBean.class, "1"); // "1" is a string value for 'sr' field which is primary key object.setObject(myjson); // myjson is a string value for 'object' field which is required realm.insert(object); } catch (JSONException e) { e.printStackTrace(); } } }); |
7. Delete data
Use below boilerplate code to delete data from the realm database.
Use below boilerplate code to delete data from the realm database.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | realm.executeTransaction(new Realm.Transaction() { @Override public void execute(Realm realm) { try { RealmResults<RealmBean> results; results = realm.where(RealmBean.class).findAll(); for(int i = 0 ; i < results.size(); i++){ JSONObject jsonObject1 = new JSONObject(results.get(i).getObject()); if(jsonObject1.toString().equals(myjson)){ // myjson is a string value for 'object' field which I want to delete results.get(i).deleteFromRealm(); } } }catch (Exception e){ e.printStackTrace(); } } }); |
Thanks for reading! Happy coding :)
An international data SIM card is a SIM card that can be used to access data services while traveling outside of the country where the SIM card was purchased. Unlike a traditional SIM card, which is tied to a specific carrier and only works in one country, an international data SIM card allows users to access data services across multiple countries and carriers.
ReplyDelete